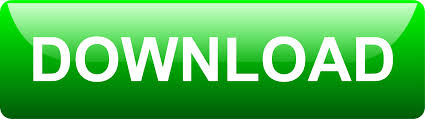
With that change, the code above decodes the message quickly.įurther improvements are possible, but more complicated, and won't be drop-in replacements. the first instruction encrypts the byte as C: using the RSA encryption mathematical expression ( stands for exponentional, and for modulus in Python programming language) the second decrypts C to get M back: using the RSA decryption mathematical expression. So decrypt can be written as: def decrypt(kenc, d, n): You could implement it yourself, but Python handily provides a built-in function for this: pow(x, e, n) There is a simple remedy: use modular exponentiation, which keeps the size of the numbers that it is working with low throughout the whole calculation by reducing modulo n as it goes along. Python's built-in pow can do modular exponentiation, so that will be our decryption function: import sys n 65354147 e 13 d 35181901 def decrypt (c): return pow (c, d, n) with open (sys.argv 1, 'r') as f: message '' for line in f: message + chr (decrypt (int (line. This does not finish in a reasonable time. Key = RSA.generate(1024, random_generator) For example, trying it out with 1024bit RSA (the lowest setting!): import Crypto

Kenc**d is in general a very big number that takes a long time to compute, and then it takes a long time again to reduce it modulo n. There is a serious problem with this implementation: it computes kenc**d. In this case though, there is a much more efficient solution that is about equally simple, and is probably sufficient.
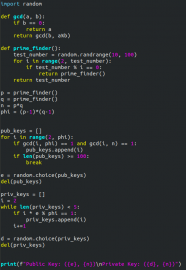
Usually the most efficient way to perform a non-trivial task is not also the simplest way to do it. You should investigate modular exponentiation. Osiris at 20:27 You don't actually need to calculate that big power. You probably need a bigint library, or you take a look at modular exponentiation. Simple does not mean fast, so you cannot judge performance based on how simple the implementation looks. 1 pow and fmod are for double, do not use floating point functions when you calculate with integers.
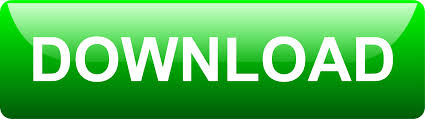